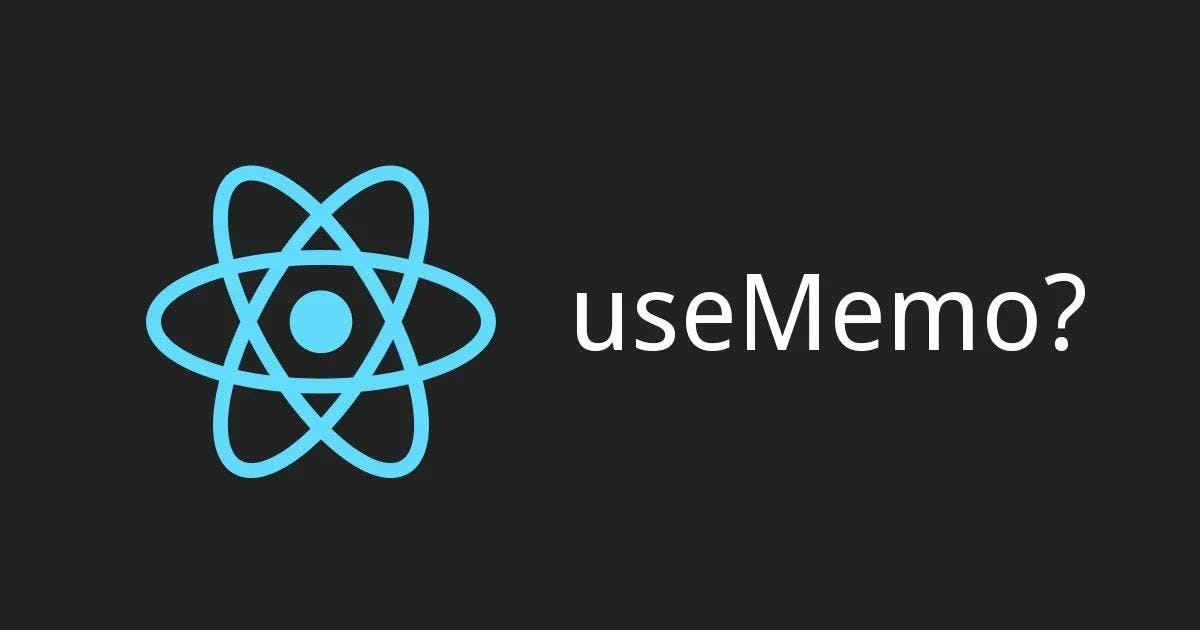
How to Use the useMemo Hook in React
March 4, 2023

Ivaylo Tsochev
If you’re looking for a way to improve the performance of your React components, look no further than the useMemo hook.
React
Development
If you’re looking for a way to improve the performance of your React components, look no further than the useMemo hook. This handy little hook allows you to memoize (or cache) the return value of a function so that it is only calculated once, even if it is called multiple times. In this blog post, we’ll take a look at some tips and tricks for using the useMemo hook in your own React components.
So, what exactly is memoization?
Memoization is an optimization technique that is used to avoid calculating the same value multiple times. When you memoize a function, you store the result of that function in a cache so that it can be retrieved later. This can be especially useful for expensive operations such as database queries or image processing.
One common use case for the useMemo hook is to memoize the return value of a function that is called on each render.
For example, let’s say you have a component that fetches data from an API on each render. This can be slow and cause performance issues, especially if the data doesn’t change often. To solve this problem, you can use the useMemo hook to memoize the return value of the function that fetches the data. This way, the data is only fetched once and then stored in a cache.
Here’s a simple example of how to use the useMemo hook:
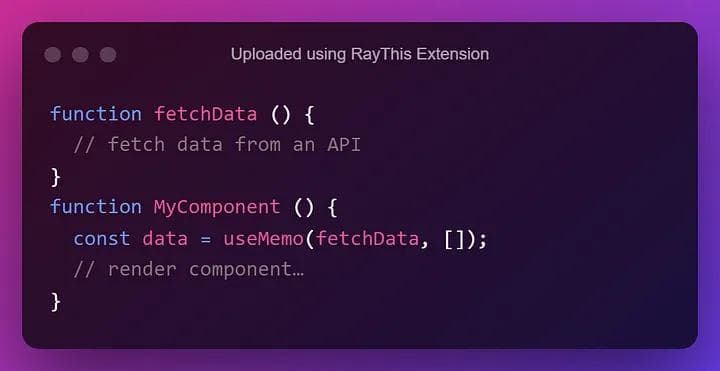
In the example above, we’re using the useMemo hook to memoize the return value of the fetchData function. The fetchData function is only called once, on the initial render of the component. After that, the data is retrieved from the cache.
It’s important to note that you should only use the useMemo hook if you actually need to memoize a value. If you don’t need to memorize a value, then using the useMemo hook will just add unnecessary overhead to your component.
Another use case for the useMemo hook is to memoize the return value of a function that depends on props.
For example, let’s say you have a component that renders a list of items. Each item in the list has a unique id, and each time the id changes, the component needs to fetch new data for that item.
To avoid fetching the same data multiple times, you can use the useMemo hook to memoize the return value of the function that fetches the data.
Here’s a simple example of how to do this:
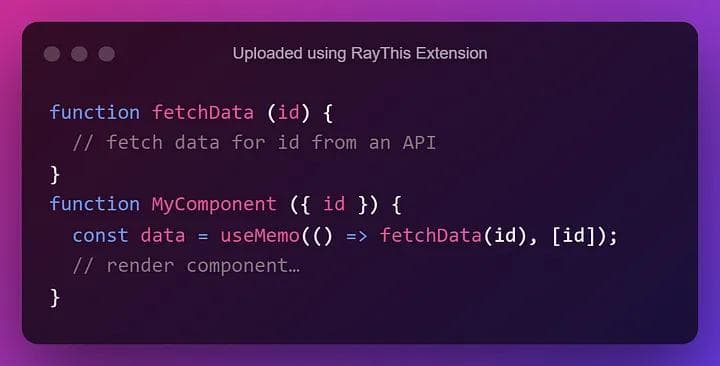
In the example above, we’re using the useMemo hook to memoize the return value of the fetchData function. The fetchData function is only called when the id prop changes. After that, the data is retrieved from the cache.
That’s all there is to using the useMemo hook! By using this handy little hook, you can improve the performance of your React components by avoiding unnecessary calculations. Give it a try in your own components and see how it works for you.
Do you have any tips or tricks for using the useMemo hook? Share them in the comments below!